Getting Started with Terraform
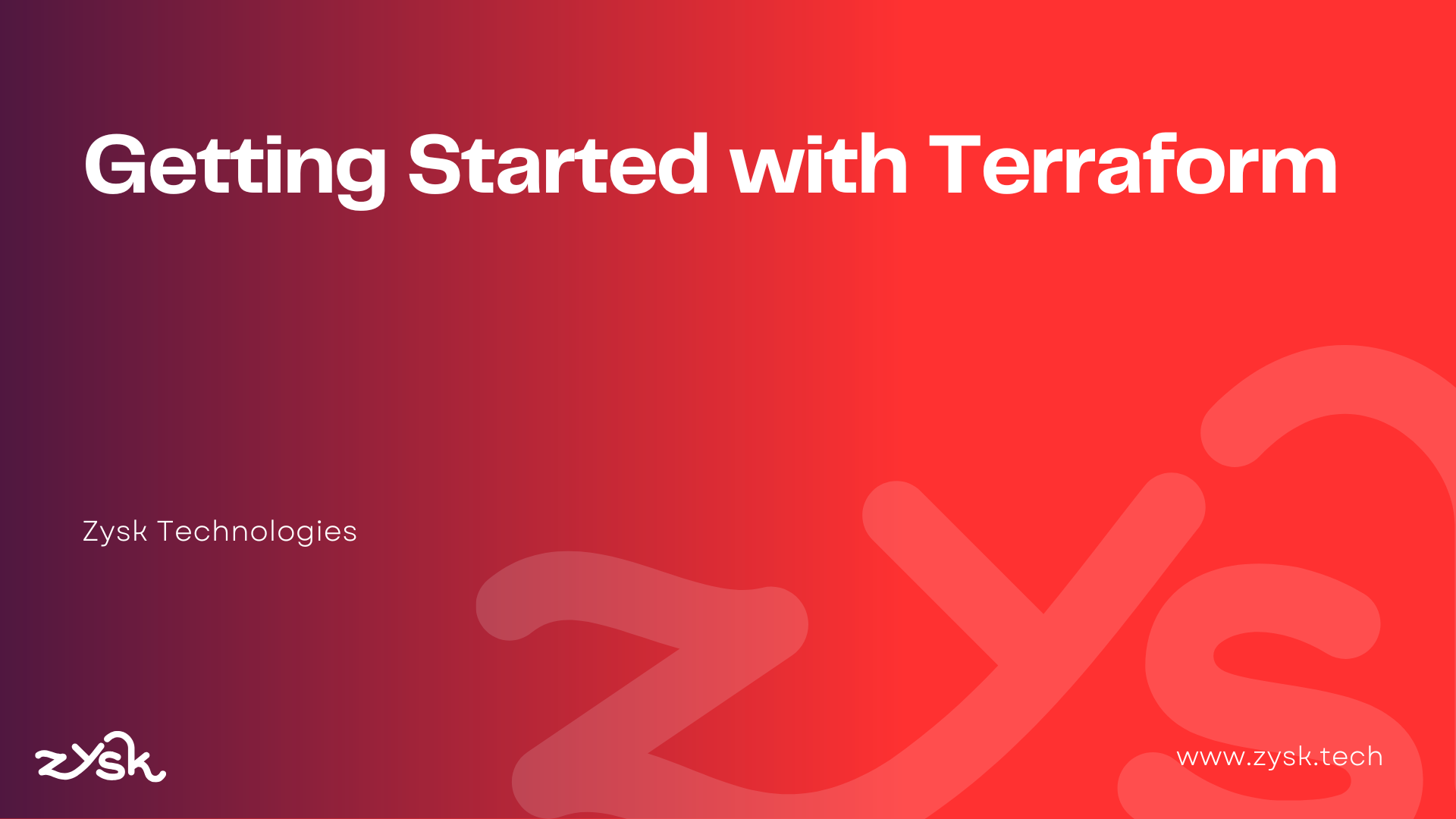
Terraform lets you manage your server architecture using code, a concept called Infrastructure as Code (IaC). It’s declarative, meaning you don’t have to code each step; simply define the final setup you want, and Terraform takes care of the rest.
It lets you build, modify, and update and delete your cloud infrastructure using code.
Why Terraform?
- Multi-Cloud Support
Works with all the major providers like AWS, GCP, Azure, and more, so you can manage everything from one place. - Stateful Management
Terraform remembers your infrastructure’s state, so updates are smoother, and everything stays in sync. - Version Control
You can keep Terraform configs in Git, making it easy to work with your team and track every change. - Declarative Approach
Just tell Terraform what you want it handles adding, deleting, and updating automatically. - Less Manual Work
Once you’ve set it up, you can reuse it anytime. No more clicking around for every setup, which saves time (and sanity). - Cost-Efficient
Terraform makes it easy to start and stop resources, so you’re only paying for what you actually need. - Disaster Recovery
If things go south, you can bring everything back with just a command or two. Fast recovery without the stress. - Fewer Errors
Writing your setup as code cuts down on manual steps (and mistakes), which means more reliable deployments overall.
Prerequisites
I prefer using winget
, a package manager for Windows similar to apt
on Ubuntu. Below are the winget
commands for quick installation. Alternatively, you can use the linked resources:
- Terraform: 📎Download Link
# winget command (skip if you already downloaded using the link)
winget install -e --id Hashicorp.Terraform
Once Terraform is installed, you can verify with the command
terraform --version
you should see an output as
Terraform v1.9.8
on windows_amd64
- AWS CLI: 📎Download Link
Since we’re working with AWS, I’m installing the AWS CLI to interact with our AWS services directly from the command line. This makes it easier to configure, manage, and verify resources as we go along.
# winget command (skip if you already downloaded using the link)
winget install -e --id Amazon.AWSCLI
Configuring AWS
After installation, use the following command to configure AWS:
aws configure
This will prompt you to enter your AWS Access Key, Secret Key, default region, and output format. Enter these details to complete the setup. For a more detailed guide, see 📎this tutorial on Medium.
To verify your configuration, navigate to the .aws
directory and view the credentials file:
cd .aws
cat credentials # On Linux/Mac
or on Windows:
cd .aws
notepad credentials
You should see a [default]
section with the aws_access_key_id
and aws_secret_access_key
you entered:
[default]
aws_access_key_id = YOUR_ACCESS_KEY
aws_secret_access_key = YOUR_SECRET_KEY
Remember the [default]
profile—we’ll be using it in a later phase.
Writing Your First Terraform Code
Now that everything is set up, let’s write our first Terraform code!
Start by creating a new folder
for your project, then open it in your preferred code editor (I’m using Visual Studio Code here). Inside this folder, create a file with the .tf
extension this is where you’ll write your Terraform configurations. For better coding support, like IntelliSense, I recommend installing the Terraform extension in Visual Studio Code to make writing and troubleshooting your code even easier.
extension Name: HashiCorp Terraform
Id: hashicorp.terraform
Description: Syntax highlighting and autocompletion for Terraform
Version: 2.33.0
Publisher: HashiCorp
VS Marketplace Link: https://marketplace.visualstudio.com/items?itemName=HashiCorp.terraform
Let's Try Creating a Simple EC2 Instance Using Terraform
I’ve added a code snippet below to set up a basic EC2 instance. You can adjust it to fit your needs or check the 📎Terraform Documentation to create your own configuration. Just pick your provider for specific references.
provider "aws" {
profile = "default"
region = "ap-south-1"
}
resource "aws_instance" "terraform-demo" {
ami = "ami-08bf489a05e916bbd"
instance_type = "t2.micro"
tags = {
Name = "TestTerraformInstance"
}
}
Code Breakdown
- Provider block
provider "aws"
: Defines AWS as the provider here, but you could switch to any cloud provider that Terraform supports.profile = "default"
: Tells Terraform to use the default AWS profile on your system. Remember, we saw[default]
in thecredentials
file when we usedcat
ornotepad
to check the AWS configuration.region = "ap-south-1"
: Specifies the AWS region for this deployment (in this case, it’s Mumbai, Asia Pacific).
- Resource block
resource "aws_instance" "terraform-demo"
: Here,resource
tells Terraform that we’re referring to an AWS resource, andaws_instance
is the identifier for an EC2 instance in AWS."terraform-demo"
is just the name we’re giving it within Terraform so we can refer to it easily in our configuration.ami = "ami-08bf489a05e916bbd"
: Sets the Amazon Machine Image (AMI) ID, which specifies the OS for this instance.instance_type = "t2.micro"
: Defines the instance type ast2.micro
, which is a general-purpose, low-cost instance.tags
: Lets you tag the instance with metadata; here, it’s named"TestTerraformInstance"
so it’s easier to identify."
.
Now that we’ve finished the configuration, let’s execute it and see if it works! Remember, we installed Terraform, which gives us a few key commands. You can check them all by running:
terraform --help
Here are some main commands to know:
Main commands:
init Prepare your working directory for other commands
validate Check whether the configuration is valid
plan Show changes required by the current configuration
apply Create or update infrastructure
destroy Destroy previously-created infrastructure
To get started, we first need to run:
terraform init
This command will set up the necessary configurations for the AWS provider, creating a .terraform
folder with files like:
terraform
┣ .terraform
┃ ┗ providers
┃ ┃ ┗ registry.terraform.io
┃ ┃ ┃ ┗ hashicorp
┃ ┃ ┃ ┃ ┗ aws
┃ ┃ ┃ ┃ ┃ ┗ 5.75.1
┃ ┃ ┃ ┃ ┃ ┃ ┗ windows_amd64
┃ ┃ ┃ ┃ ┃ ┃ ┃ ┣ LICENSE.txt
┃ ┃ ┃ ┃ ┃ ┃ ┃ ┗ terraform-provider-aws_v5.75.1_x5.exe
┣ .terraform.lock.hcl
┣ main.tf
┣ terraform.tfstate
┗ terraform.tfstate.backup
Once the command finishes executing, you should see something like this:
Terraform has been successfully initialized!
You may now begin working with Terraform. Try running "terraform plan" to see
any changes that are required for your infrastructure. All Terraform commands
should now work.
If you ever set or change modules or backend configuration for Terraform,
rerun this command to reinitialize your working directory. If you forget, other
commands will detect it and remind you to do so if necessary.
After this setup, you can run:
terraform apply
This command will prompt for the confirmation enter yes
and it will apply your configuration and create the new instance on AWS. If you want to make any changes later, just edit the config and run terraform apply
again the changes will be applied to the instance automatically without any extra effort.
To take down the instance, just run:
terraform destroy
Boom! The instance we created will be deleted. It’s really that simple!
Conclusion
I hope this blog gave you a solid introduction to Terraform and got you thinking about all the possibilities. With Terraform, the only limit is your imagination! It’s a powerful tool in the DevOps toolkit that makes managing infrastructure simpler and more efficient. And with the rise of AI, writing Terraform scripts will only get easier. You can even use AI to help generate configurations based on your specific application needs, making automation faster and smarter. Happy building and automating!